Overview / Getting Started
Embedded apps are a way to load your data into the Follow Up Boss UI. For more basic information, see our basic overview article. If you're ready to start building embedded apps, you will need to request access to this feature.
You will be creating a secure https URL that will be loaded into an iframe. This page needs to include the following JavaScript snippet:
<script type="text/javascript" src="https://eia.followupboss.com/embeddedApps-v1.0.0.js"></script>
We will call this URL with two query string parameters, context and signature. The context helps you understand what account, user and person is being discussed. The signature is a way to verify that the request is from us.
Context
Your URL needs to accept a context query param. It's a base64 encoded string that you can decode to understand the topic. Here is an example what that context will look like:
URL Safe Base64 Decode
For languages like Ruby, make sure to use the urlsafe_decode64 method. Otherwise you might get some invalid parsing.
{
"example": true,
"debugState": "working",
"context": "person",
"account": {
"id": 123456789,
"domain": "example",
"owner": {
"name": "John Doe",
"email": "[email protected]"
}
},
"person": {
"id": 123,
"firstName": "Melissa",
"lastName": "Hartman",
"emails": [
{
"value": "[email protected]",
"type": "home",
"status": "Not Validated",
"isPrimary": 1
}
],
"phones": [
{
"value": "(888) 555-1234",
"normalized": "8885551234",
"type": "mobile",
"status": "Not Validated",
"isPrimary": 1
}
],
"stage": {
"id": 12,
"name": "Active Client"
}
},
"user": {
"id": 2,
"name": "Louis Tully",
"email": "[email protected]"
}
}
eyJleGFtcGxlIjp0cnVlLCJkZWJ1Z1N0YXRlIjoid29ya2luZyIsImNvbnRleHQiOiJwZXJzb24iLCJhY2NvdW50Ijp7ImlkIjoxMjM0NTY3ODksImRvbWFpbiI6ImV4YW1wbGUiLCJvd25lciI6eyJuYW1lIjoiVG9tIE1pbmNoIiwiZW1haWwiOiJ0b20ubWluY2hAZXhhbXBsZS5jb20ifX0sInBlcnNvbiI6eyJpZCI6MTIzLCJmaXJzdE5hbWUiOiJNZWxpc3NhIiwibGFzdE5hbWUiOiJIYXJ0bWFuIiwiZW1haWxzIjpbeyJ2YWx1ZSI6Im0uaGFydG1hbkBleGFtcGxlLmNvbSIsInR5cGUiOiJob21lIiwic3RhdHVzIjoiTm90IFZhbGlkYXRlZCIsImlzUHJpbWFyeSI6MX1dLCJwaG9uZXMiOlt7InZhbHVlIjoiKDg4OCkgNTU1LTEyMzQiLCJub3JtYWxpemVkIjoiODg4NTU1MTIzNCIsInR5cGUiOiJtb2JpbGUiLCJzdGF0dXMiOiJOb3QgVmFsaWRhdGVkIiwiaXNQcmltYXJ5IjoxfV19LCJ1c2VyIjp7ImlkIjoxLCJuYW1lIjoiSm9obiBEb2UiLCJlbWFpbCI6ImouZG9lQGV4YW1wbGUuY29tIn19
Map Account & User Identification
Your application likely needs to be able to map your known accounts and users to Follow Up Boss accounts and users. To do this, you should look at using the /identity API endpoint.
Debug Contexts
To better help in your development, we have created some sample context states that your app can respond to. This will help us to evaluate your app, and it'll make it easier for you to test our your application during development.
debugState & example
debugState
andexample
attributes are only passed in during previewing of the app. Live versions will not have these attributes. It's also used for us to better monitor your app. More on that below.
When you're filling out your app details, you'll be able to preview different context states.
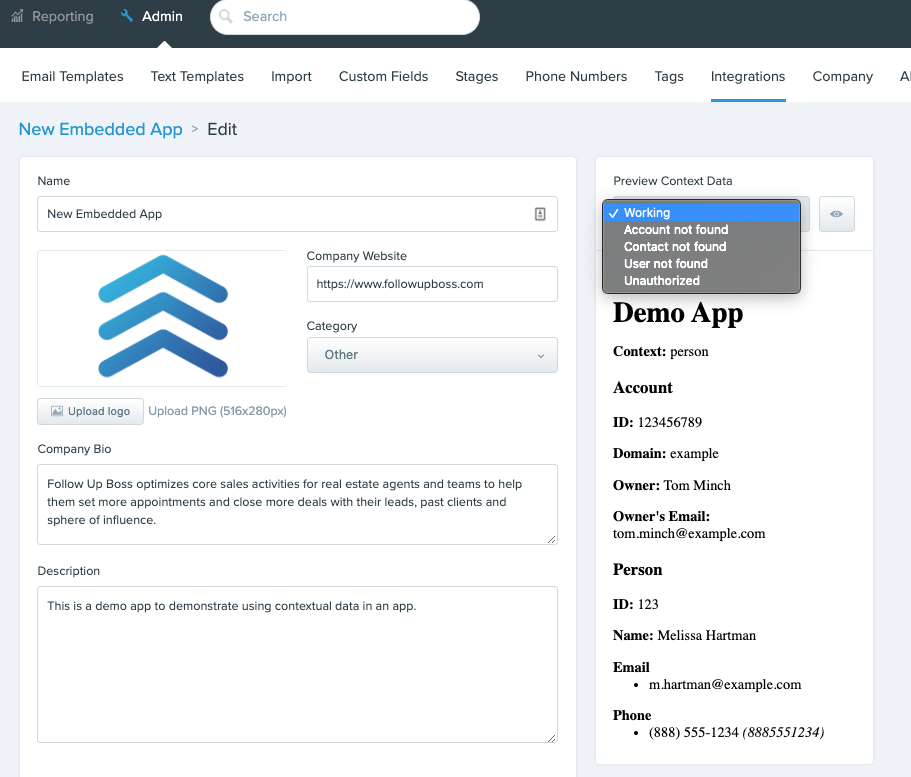
Preview context data with debug state
These debug states are just for simulating various response formats. It's not required you have a specific UI for each of them. These different states allow you to simulate the UI states that your app needs. We will require the working
state functions, but your app might not need a user_not_found
state.
- working: This is the most common state, and the main reason people will be enabling your app. This debug state is required to demonstrate.
- account_not_found: If you fail to match up the provided account, this is your opportunity to help get them set up. You can provide links about how to get fully integrated with your system.
- user_not_found: If you need to also verify the user, this is a way for you to test against this state.
- person_not_found: If you don't have any information on the current person, then you would want to respond saying something to the effect of "Person not found in our system".
- unauthorized: You might just want to inform people they don't have access.
Security
We take security seriously, so we've taken some precautions to help that we both protect our data.
Verify the request
Each request, we pass along a signature
query param. This string you can use to verify the request is from us. When you create your embedded app, you'll be provided with a secret key.
To create the signature, you will need to generate an hmac using sha256 encoding, the base64 context string (context query string) and the secret key we provided you. Here are some examples:
function isFromFollowUpBoss(string $context, string $signature): boolean {
$calculated = hash_hmac('sha256', $context, YOUR_SECRET_KEY);
return $signature === $calculated;
}
if (isFromFollowUpBoss($_GET['context'], $_GET['signature'])) {
// do something
}
const crypto = require('crypto');
function isFromFollowUpBoss(context, signature) {
const calculated = crypto
.createHmac('sha256', process.env.SECRET_KEY)
.update(context)
.digest('hex');
return calculated === signature;
}
if (isFromFollowUpBoss(req.query.context, req.query.signature)) {
// do something
}
iFrame sandboxing
We sandbox the iframe to prevent basic security issues. Because of this, you will need to remove the X-Frame-Options
from your header. If this is set at all, it'll automatically fail the CORS policy.
Older Browsers & IE11
Because older browser don't support modern CORS practices, we disable the iFrame entirely. Our current browser support is the latest versions of Edge, Safari, Chrome and Firefox.
Submitting Your Application
Once your application is ready, you've filled out all of the required information within Follow Up Boss, and tested how it responds to different states, you may submit your application for review.
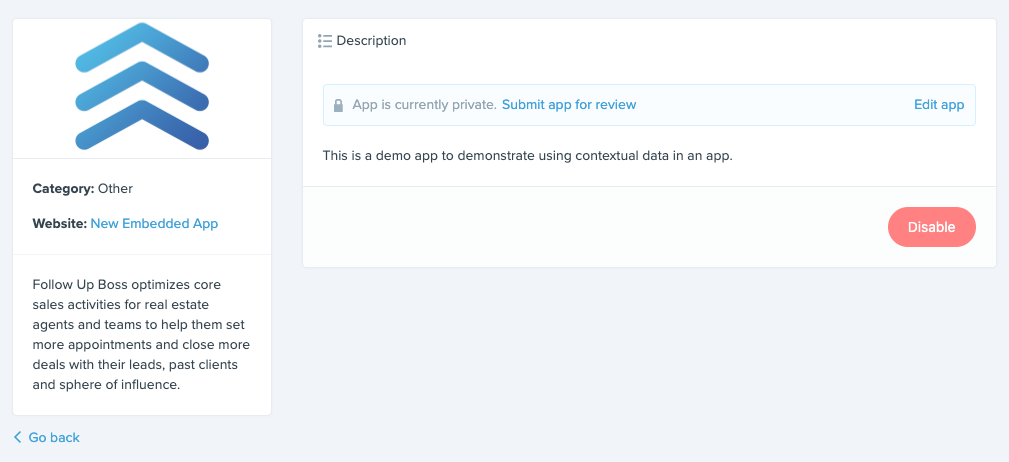
Submit for review
You'll see your app's status on the details page. Once you're ready to publish the application for Follow Up Boss accounts to use, hit the "Submit app for review" button. Follow Up Boss will be alerted that your app is ready for review.
What We're Looking For
When reviewing your application, Follow Up Boss will ensure the basics are set like a descriptive app name, description, appropriate thumbnail, URL, and company bio and company website. From there, we'll actually preview the application ourselves and test the various debug states to ensure it loads properly and presents well. Change requests or feedback will be communicated back to you.
Once everything looks good, we'll approve the application and it'll be available to Follow Up Boss customers to enable for their accounts.
App Styling
We do ask that you try and match our styles to some degree. The more it looks and feels like our app, the better the user experience is. At this moment, we don't have a strict guide. However, we may ask of you to adjust styles.
Monitoring and Error Handling
Errors should be properly recorded and handled on your system. We will periodically ping the app's URL with the test context and record any errors on our side. If we detect too many errors or too frequent of errors, we will contact you to resolve any problems.
Further Assistance
If you have any further questions regarding embedded app creation or need assistance with the approval process, please reach out to our API Questions Inbox.